Understanding DataFrame Shape In Python: A Comprehensive Guide
DataFrame shape is a crucial concept in data manipulation and analysis, especially when using libraries like Pandas in Python. Understanding the shape of a DataFrame allows data scientists and analysts to grasp the dimensions of their dataset, which is vital for effective data processing and visualization. In this article, we will explore what DataFrame shape is, its importance, and how to work with it in Python, particularly using the Pandas library.
As we delve deeper into this topic, we'll provide insights into the different attributes of a DataFrame, demonstrate how to access and interpret its shape, and discuss practical applications of this knowledge. Moreover, we will also highlight the significance of DataFrame shape in ensuring data integrity and preparing datasets for analysis.
By the end of this article, you will have a thorough understanding of DataFrame shape, enabling you to leverage this knowledge in your data analysis projects effectively. So, let's get started!
Table of Contents
- What is DataFrame Shape?
- Importance of DataFrame Shape
- Accessing DataFrame Shape in Pandas
- Examples of DataFrame Shape
- Modifying DataFrame Shape
- Common Issues with DataFrame Shape
- Visualization and DataFrame Shape
- Conclusion
What is DataFrame Shape?
The shape of a DataFrame refers to its dimensions, specifically the number of rows and columns it contains. In Python's Pandas library, the shape is represented as a tuple, typically in the format (rows, columns). For example, a DataFrame with 100 rows and 5 columns will have a shape of (100, 5).
Understanding the shape of a DataFrame is essential for several reasons:
- It helps you understand the size of your dataset.
- It enables you to verify data integrity by checking for unexpected changes in dimensions.
- It is critical for determining the appropriate statistical techniques and visualizations to apply.
Importance of DataFrame Shape
Knowing the shape of a DataFrame is not just a technical detail; it has significant implications for data analysis. Here are some key reasons why understanding DataFrame shape is important:
- Data Validation: Ensuring the expected number of rows and columns helps identify data issues early in the analysis process.
- Memory Management: Understanding the size of your DataFrame helps optimize memory usage, especially with large datasets.
- Efficient Operations: Certain operations in Pandas require specific DataFrame shapes (e.g., merging, joining, or reshaping data).
Accessing DataFrame Shape in Pandas
In Pandas, accessing the shape of a DataFrame is straightforward. You can use the `.shape` attribute, which returns the shape as a tuple. Here's how to do it:
# Importing Pandas library import pandas as pd # Creating a sample DataFrame data = { 'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35], 'City': ['New York', 'Los Angeles', 'Chicago'] } df = pd.DataFrame(data) # Accessing the shape of the DataFrame dataframe_shape = df.shape print(dataframe_shape) # Output: (3, 3)
The output (3, 3) indicates that the DataFrame has 3 rows and 3 columns.
Examples of DataFrame Shape
Let’s look at a couple of examples to illustrate how to work with DataFrame shape in different scenarios:
Example 1: Basic DataFrame Shape
# Creating a DataFrame with different data types data = { 'Product': ['Laptop', 'Smartphone', 'Tablet'], 'Price': [1000, 500, 300], 'In_Stock': [10, 20, 30] } df_products = pd.DataFrame(data) # Checking the shape print(df_products.shape) # Output: (3, 3)
Example 2: Large DataFrame Shape
# Creating a large DataFrame import numpy as np # Creating a DataFrame with 1000 rows and 5 columns large_data = np.random.rand(1000, 5) df_large = pd.DataFrame(large_data, columns=['A', 'B', 'C', 'D', 'E']) # Checking the shape print(df_large.shape) # Output: (1000, 5)
Modifying DataFrame Shape
Sometimes, you may need to modify the shape of a DataFrame to meet the requirements of your analysis. Here are some common methods to do this:
Adding Rows
You can add rows to a DataFrame using the `append()` method or `concat()` function:
# Adding a new row to the DataFrame new_row = pd.DataFrame({'Product': ['Headphones'], 'Price': [100], 'In_Stock': [50]}) df_products = df_products.append(new_row, ignore_index=True) # Checking the new shape print(df_products.shape) # Output: (4, 3)
Removing Rows
To remove rows, you can use the `drop()` method:
# Removing a row by index df_products = df_products.drop(0) # Removes the first row # Checking the new shape print(df_products.shape) # Output: (3, 3)
Adding Columns
You can add columns to a DataFrame by simply assigning a new column name:
# Adding a new column df_products['Rating'] = [4.5, 4.0, 4.2] # Checking the new shape print(df_products.shape) # Output: (3, 4)
Removing Columns
To remove a column, you can use the `drop()` function with the `axis` parameter set to 1:
# Removing a column df_products = df_products.drop('Rating', axis=1) # Checking the new shape print(df_products.shape) # Output: (3, 3)
Common Issues with DataFrame Shape
When working with DataFrames, you may encounter several issues related to shape. Here are some common ones and how to address them:
- Unexpected Shapes: If your DataFrame shape does not match your expectations, check for issues in data loading or transformations.
- Duplicate Rows: Use the `drop_duplicates()` method to remove any unintended duplicate rows that may alter the shape.
- Missing Values: Missing values can affect the shape. Use methods like `fillna()` or `dropna()` to handle them appropriately.
Visualization and DataFrame Shape
The shape of a DataFrame plays a significant role in data visualization. Many visualization libraries, such as Matplotlib and Seaborn, require data to be in specific shapes. Here are some ways shape impacts visualization:
- Heatmaps: The shape of the DataFrame must correspond to the number of variables you want to visualize. For example, a heatmap requires a 2D array.
- Graphs: When plotting graphs, the number of data points (rows) can affect the clarity and interpretability of the graph.
- Grouping Data: Understanding shape helps in properly grouping data for visualizations that summarize or aggregate information.
Conclusion
In this comprehensive guide, we explored the concept of DataFrame shape in Python's Pandas library. Understanding the shape of a DataFrame is essential for effective data manipulation, analysis, and visualization. We discussed how to access, modify, and troubleshoot DataFrame shape, highlighting its importance
Genevieve Gregson: The Rising Star Of Australian Athletics
Caoimhe O'Neill: The Rising Star Of Irish Journalism
Understanding Dia Abrams: A Comprehensive Look At Her Life And Career
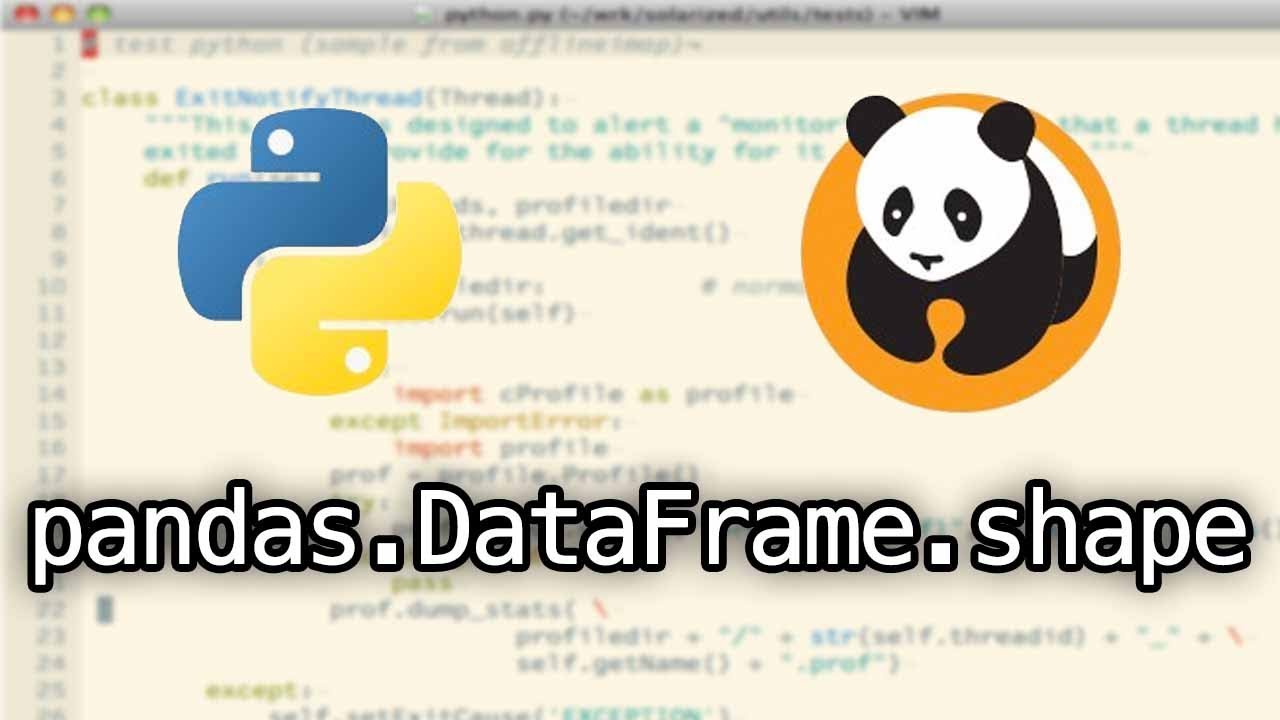
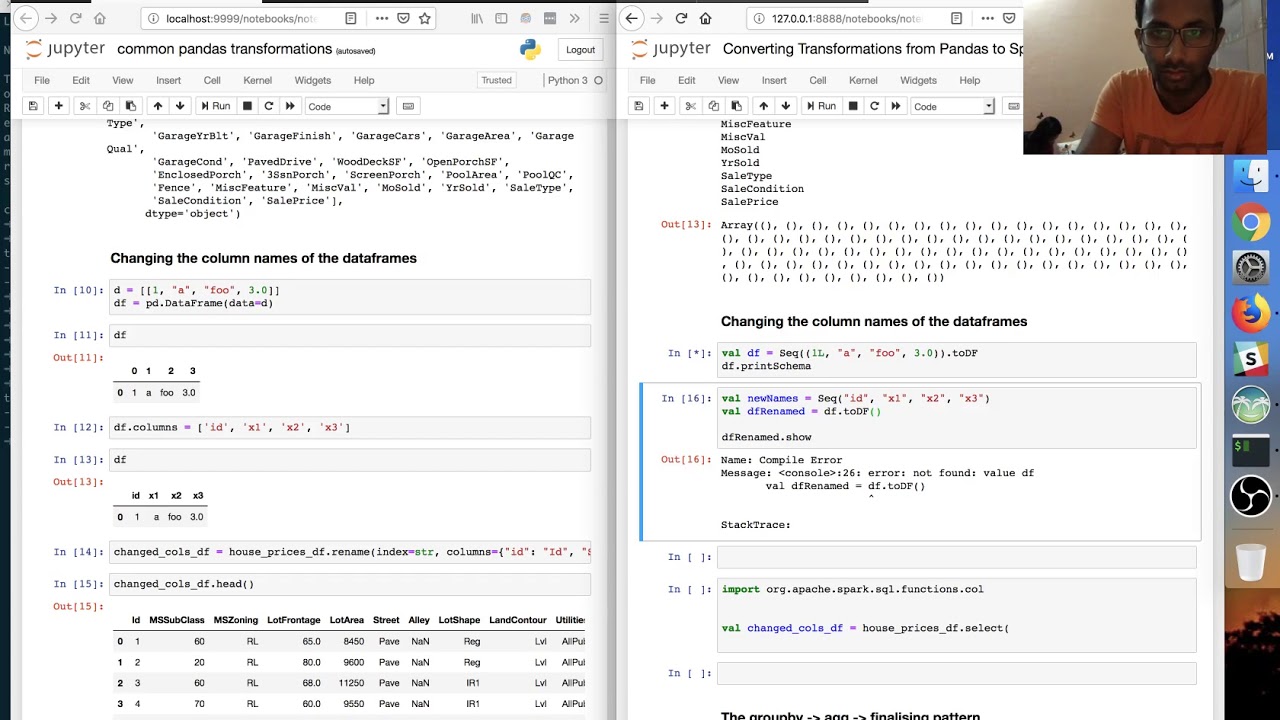
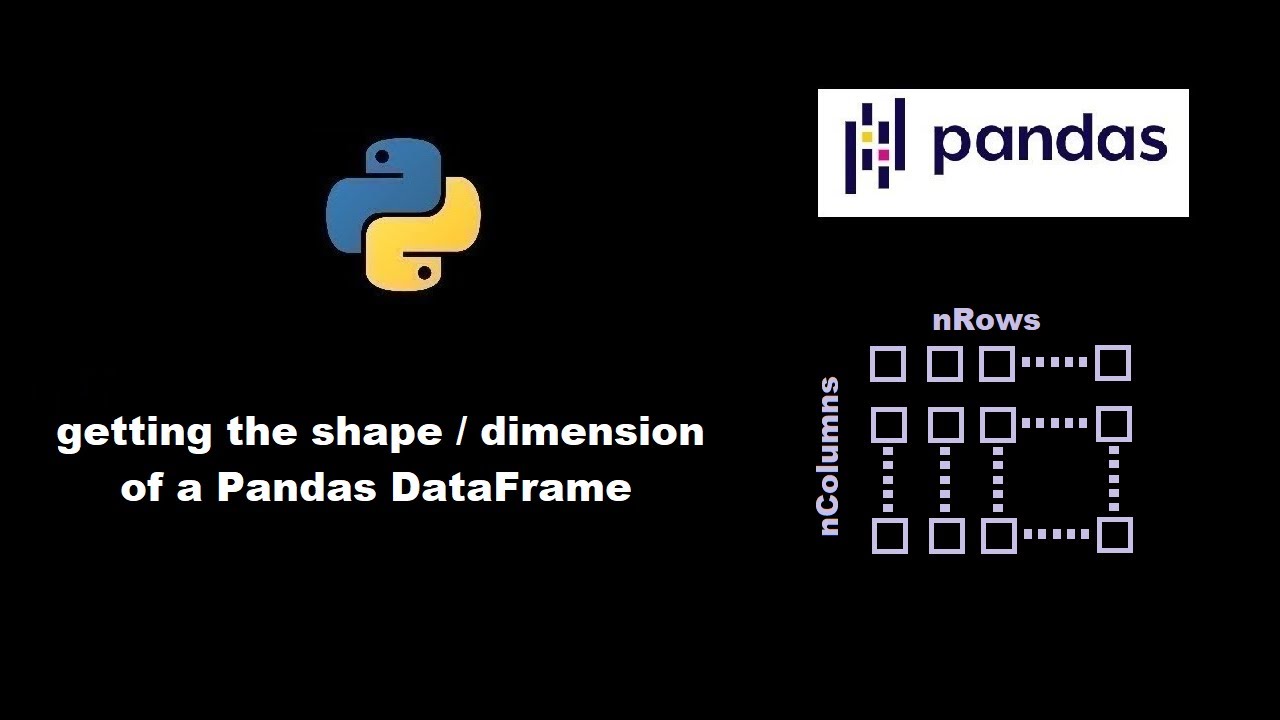