Understanding The Python String Method: Str.endswith
In the realm of programming, mastering string manipulation is crucial, and one of the essential methods in Python is the str.endswith method. This method is incredibly useful for determining if a string ends with a specified suffix, making it a valuable tool for developers. In this article, we will delve deep into the functionality of the str.endswith method, exploring its syntax, usage, and practical examples that showcase its effectiveness. Whether you are a novice programmer or a seasoned developer, understanding this method will enhance your Python string manipulation skills.
Strings are one of the most common data types in Python, and knowing how to work with them efficiently can save time and reduce errors in your code. The str.endswith method not only checks the end of a string but also supports multiple suffixes and optional start and end parameters, making it versatile for various applications. Throughout this article, we will provide detailed explanations, real-world examples, and best practices to ensure you fully grasp how to use str.endswith effectively.
By the end of this article, you will have a comprehensive understanding of the str.endswith method and how to implement it in your projects. So, let’s dive in and explore this powerful string method in Python!
Table of Contents
- What is str.endswith?
- Syntax of str.endswith
- Parameters of str.endswith
- Return Value
- Examples of str.endswith
- Common Use Cases for str.endswith
- Performance Considerations
- Conclusion
What is str.endswith?
The str.endswith
method is a built-in function in Python that checks whether a given string ends with a specific suffix or a tuple of suffixes. This method returns a boolean value: True
if the string ends with the specified suffix, and False
otherwise. This functionality is particularly useful for validating data, processing text, and managing file extensions.
Syntax of str.endswith
The syntax for the str.endswith method is as follows:
string.endswith(suffix[, start[, end]])
Here:
suffix
: This is the suffix you want to check against the string. It can be a string or a tuple of strings.start
(optional): An optional parameter that specifies the starting index from where to check the suffix.end
(optional): An optional parameter that specifies the ending index up to which to check the suffix.
Parameters of str.endswith
Let’s take a closer look at the parameters of the str.endswith method:
- suffix: The string or tuple of strings that you want to check. For example, if you want to check if a filename ends with '.txt', '.pdf', or '.docx', you can provide these as a tuple.
- start: This optional parameter defines the starting position for the check. If not specified, it defaults to the beginning of the string.
- end: This optional parameter defines the ending position for the check. If not specified, it defaults to the end of the string.
Return Value
The str.endswith method returns a boolean value:
True
: If the string ends with the specified suffix.False
: If the string does not end with the specified suffix.
Examples of str.endswith
Here are some practical examples demonstrating how to use the str.endswith method:
Example 1: Basic Usage
filename ="report.txt" print(filename.endswith(".txt")) # Output: True
Example 2: Using a Tuple of Suffixes
filename ="presentation.pdf" print(filename.endswith((".txt", ".pdf", ".docx"))) # Output: True
Example 3: Using Start and End Parameters
text ="Hello, welcome to Python programming" print(text.endswith("programming", 7, 30)) # Output: True
Common Use Cases for str.endswith
The str.endswith method has various applications in programming, including:
- Validating file extensions when processing files.
- Checking the format of strings in data validation.
- Filtering lists based on specific suffixes.
- Implementing conditional logic based on string endings.
Performance Considerations
While the str.endswith method is efficient, it’s essential to keep in mind:
- Using tuples for multiple suffix checks is generally more efficient than checking each suffix individually.
- When specifying start and end indices, ensure they are within the bounds of the string to avoid unexpected results.
Conclusion
In this article, we explored the str.endswith method in Python, its syntax, parameters, and practical use cases. Understanding this method is crucial for effective string manipulation and validation in Python programming. By leveraging str.endswith, you can enhance your code's reliability and efficiency.
Now that you have a solid understanding of the str.endswith method, we encourage you to experiment with it in your projects. Feel free to leave a comment below if you have any questions or would like to share your experiences using this method!
Exploring The Life And Career Of Lauren Glaser: A Comprehensive Guide
Mails: The Evolution And Importance Of Email Communication
Understanding The Peus Scandal: A Comprehensive Overview
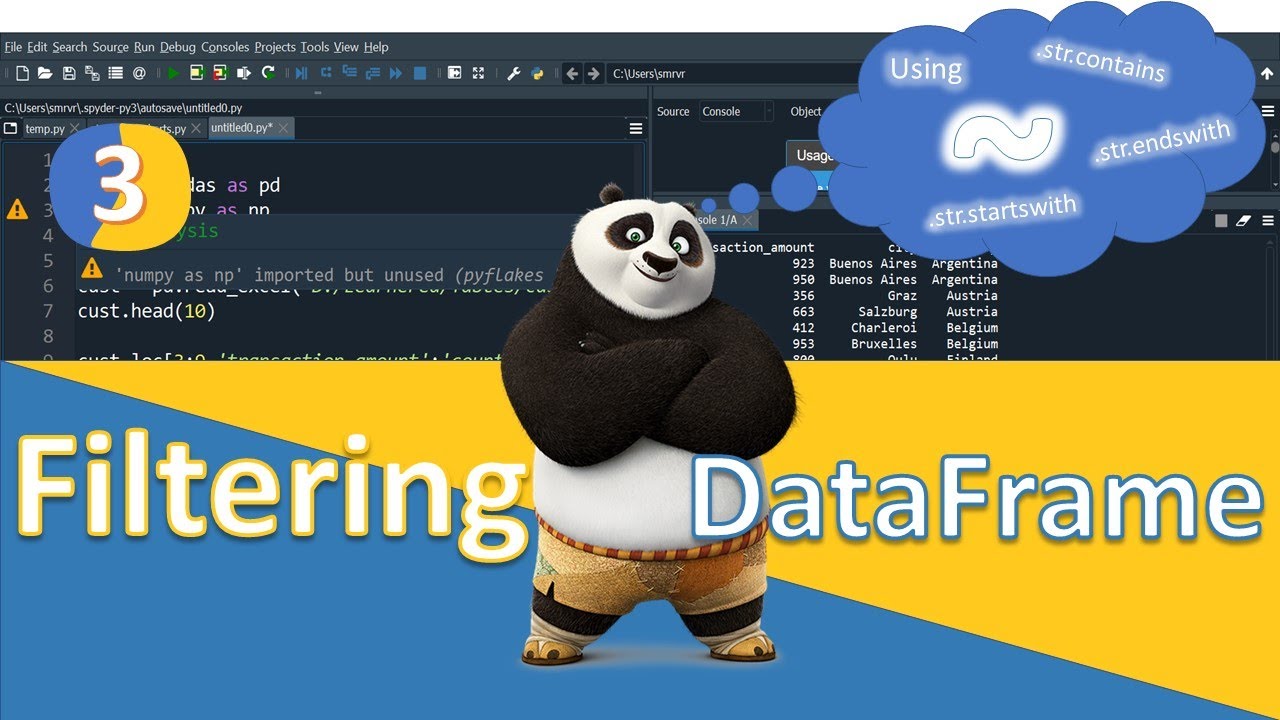
